Navigate
IoT Device Programming with JavaScript
- Versatile IoT Development: JavaScript for Connected Devices
- Prototyping IoT Projects with Raspberry Pi and JavaScript
- Exploring JavaScript Integration with Google Cloud IoT Services
- How can JavaScript libraries enhance IoT device UI/UX for end-users?
- What innovative IoT applications can be developed using JavaScript and machine learning?
- How does JavaScript enable IoT device integration with cloud platforms and APIs?
JavaScript in Google Ads and Analytics
Exploring JavaScript frameworks
JavaScript for AI-driven Web Applications
Exploring JavaScript Integration with Google Cloud IoT Services
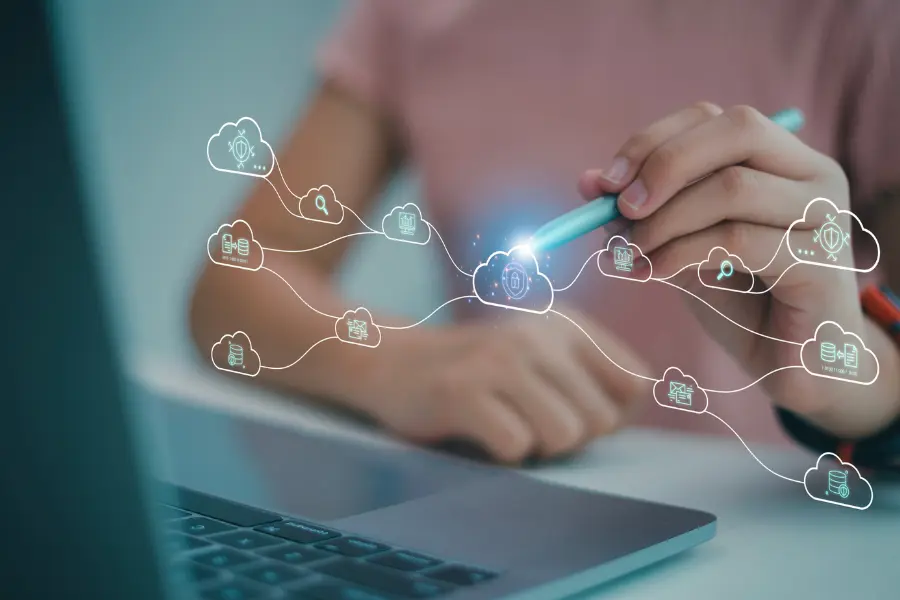
The Internet of Things (IoT) has revolutionized the way devices connect, communicate, and share data. Managing these devices and the data they provide requires efficient cloud systems. Google Cloud IoT offers a set of interconnected services for effective IoT device control and data processing. By leveraging JavaScript, particularly with Node.js, developers can efficiently utilize these services to create complex IoT applications.
Overview of Google Cloud IoT Services
Google Cloud IoT includes several essential tools:
- IoT Core: Facilitates secure connection, management, and data ingestion from IoT devices.
- Dataflow: Allows for real-time data processing.
- BigQuery: Enables powerful, scalable data analytics.
- Cloud Machine Learning Engine: Supports the deployment of machine learning models for deeper insights.
These services collectively provide a robust framework for managing the lifecycle of IoT data, from initial device connectivity to advanced data analytics and machine learning.
Getting Started with JavaScript and Google Cloud IoT Core
JavaScript, with its powerful runtime environment Node.js, is ideal for IoT development due to its asynchronous, event-driven programming capabilities. Follow these steps to integrate JavaScript with Google Cloud IoT Core.
Setup and Configuration
First, install Node.js and the Google Cloud SDK. Initialize a new Node.js project and install the required dependencies:
npm init -y npm install --save @google-cloud/iot
Authentication
Authenticate your application using a service account. Download the credentials JSON file and set the GOOGLE_APPLICATION_CREDENTIALS
environment variable:
export GOOGLE_APPLICATION_CREDENTIALS="path/to/your/service-account-file.json"
Connecting to IoT Core
Create a client to interact with IoT Core:
const { v1 } = require('@google-cloud/iot');
const client = new v1.DeviceManagerClient();
const projectId = 'your-project-id';
const location = 'your-region';
const registryId = 'your-registry-id';
async function listDevices() {
const formattedParent = client.registryPath(projectId, location, registryId);
const [devices] = await client.listDevices({ parent: formattedParent });
console.log('Devices:');
devices.forEach(device => console.log(device));
}
listDevices().catch(console.error);
Publishing Data from Devices
Devices can publish telemetry data to IoT Core using the MQTT or HTTP protocols. Using MQTT, for example, with the MQTT.js library in Node.js, you can publish data as follows:
const mqtt = require('mqtt');
const deviceId = 'your-device-id';
const mqttClientId = `projects/projectId/locations/location/registries/registryId/devices/deviceId`;
const connectionArgs = {
host: 'mqtt.googleapis.com',
port: 8883,
clientId: mqttClientId,
username: 'unused',
password: createJwt(projectId, privateKey, algorithm),
protocol: 'mqtts',
secureProtocol: 'TLSv1_2_method'
};
const client = mqtt.connect(connectionArgs);
client.on('connect', () => {
console.log('Connected');
client.publish(`/devices/deviceId/events`, 'Hello from device!');
});
Analysing IoT Data with BigQuery
Once data is ingested into Google Cloud, it can be analyzed using BigQuery. The BigQuery client library for Node.js enables this integration:
const { BigQuery } = require('@google-cloud/bigquery');
const bigQueryClient = new BigQuery();
async function queryData() {
const query = `SELECT * FROM `your-dataset.your-table` LIMIT 10`;
const [rows] = await bigQueryClient.query(query);
console.log('Query Results:');
rows.forEach(row => console.log(row));
}
queryData().catch(console.error);